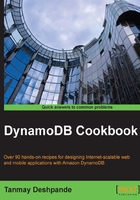
Creating a table using the AWS SDK for Java
Let's start with creating a table in DynamoDB using SDKs provided by Amazon.
Getting ready
To program various DynamoDB table operations, you can use the IDE of your choice, for example, Eclipse, NetBeans, Visual Studio, and so on. Here, we will be using the Eclipse IDE. In the previous chapter, we have already seen how to download and set up the Eclipse IDE.
How to do it…
Let's first see how to create a table in DynamoDB using the AWS SDK for Java.
You can create a Maven project and add the AWS SDK Maven
dependency. The latest version available can be found at http://mvnrepository.com/artifact/com.amazonaws/aws-java-sdk/.
Here, I will be using Version 1.9.30, and its POM looks like this:
<dependency> <groupId>com.amazonaws</groupId> <artifactId>aws-java-sdk</artifactId> <version>1.9.30</version> </dependency>
Here are the steps to create a table using the AWS SDK for Java:
- Create an instance of the
DynamoDB
class and instantiate with the AWSAccess Key
andSecret Key
credentials. To see the various ways on how to set credentials, you can refer to link: http://docs.aws.amazon.com/AWSSdkDocsJava/latest/DeveloperGuide/credentials.html:DynamoDB dynamoDB = new DynamoDB(new AmazonDynamoDBClient(new ProfileCredentialsProvider()));
- Next, we need to create the
AttributeDefinition
andKeySchema
elements in order to specify the keys. Here, we will create a similar table, that is, theproduct
table, which we created earlier using the AWS console:ArrayList<AttributeDefinition> attributeDefinitions = new ArrayList<AttributeDefinition>(); attributeDefinitions.add(new AttributeDefinition().withAttributeName("id").withAttributeType("N")); attributeDefinitions.add(new AttributeDefinition().withAttributeName("type").withAttributeType("S")); ArrayList<KeySchemaElement> keySchema = new ArrayList<KeySchemaElement>(); keySchema.add(new KeySchemaElement().withAttributeName("id").withKeyType(KeyType.HASH)); keySchema.add(new KeySchemaElement().withAttributeName("type").withKeyType(KeyType.RANGE));
- Now, instantiate
CreateTableRequest
by providing inputs, such as the table name, hash and range keys, and provisioned throughput. Here, we will create a table with the nameproductTableJava
, and we will provide the read and write capacity units as one each:CreateTableRequest request = new CreateTableRequest() .withTableName("productTableJava") .withKeySchema(keySchema) .withAttributeDefinitions(attributeDefinitions) .withProvisionedThroughput( new ProvisionedThroughput().withReadCapacityUnits(1L).withWriteCapacityUnits(1L));
- Now, invoke the
createTable
method of theDynamoDB
class, which we instantiated earlier to actually create the table. Generally, it takes some time to see the table we created getting active, so thewaitForActive
method waits until the table is active and ready to use. Now, if we execute the method, you will see that the table has been created. You can verify this from your AWS console:Table table = dynamoDB.createTable(request); table.waitForActive();
- By default, if you do not specify in which AWS region you need to create your
DynamoDB
table, it creates it inN
.Virginia
. If you need to create a table in a specific region, then you can set it at the time of theAmazonDynamoDBClient
initiation, as follows:AmazonDynamoDBClient client = new AmazonDynamoDBClient( new ProfileCredentialsProvider()); client.setRegion(Region.getRegion(Regions.US_EAST_1)); DynamoDB dynamoDB = new DynamoDB(client);
How it works…
The AWS SDK for Java calls the API to provision the table as per the details we have provided. To authenticate the requests, AWS checks the access key and secret key that you provided at the time of API invocations.