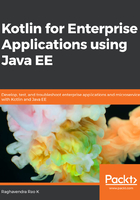
varargs and spread
Kotlin supports a variable number of arguments. vararg is the keyword that is used for a variable number of arguments. Using vararg, we can pass multiple arguments to a function.
Let's see an example of 7a_VarArgs.kts:
fun findMaxNumber(vararg numbers : Int) =
numbers.reduce { max , e -> if(max > e) max else e }
println(findMaxNumber(1,2,3,4))
The output is as follows:
The findMaxNumber() function takes a variable number of arguments and finds the maximum. We invoked reduce on the numbers and wrote a lambda expression. In Kotlin, a lambda is specified using curly braces.
If we have an array of numbers, we can invoke the findMaxNumber() function using the spread operator without changing the function argument type.
Consider the following code for 7b_Spread.kts:
fun findMaxNumber(vararg numbers : Int) =
numbers.reduce { max , e -> if(max > e) max else e }
val numberArray = intArrayOf(25,50,75,100)
println(findMaxNumber(*numberArray))
The output is as follows:
Note that * acts as a spread operator here. We can combine this with discrete values, as shown in the following 7c_Spread file.
Consider the code for 7c_Spread.kts:
fun findMaxNumber(vararg numbers : Int) =
numbers.reduce { max , e -> if(max > e) max else e }
val numberArray = intArrayOf(25,50,75,100)
println(findMaxNumber(4,5,*numberArray,200, 10))
The output is as follows: