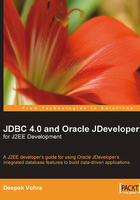
Example Connection using a JDBC 4.0 Driver
We will connect with a database using a JDBC 4.0 driver. We will use Java DB as the example database. Java DB is Sun's version of the open-source Apache Derby database. Java DB is a lightweight (only 2MB), yet fully transactional, secure, and standards-based component. It also supports the SQL, JDBC, and Java EE standards. Java DB is a 100% Java technology database, and since Java is portable across platforms, Java DB can be run on any platform and its applications can be migrated to other open standard databases. Java DB is packaged with JDK 6. Therefore, all that is required to install Java DB is to install JDK 6. We connect with Java DB database using the JDBC 4.0 driver. Create a Java application, JDBCConnection.java
, in a directory, C:/JavaDB
, and add the directory to the CLASSPATH
system environment variable. Java DB can be started in the embedded mode or as network server. Embedded mode is used to connect to the Java DB from a Java application running in the same JVM as the Java DB database. Java DB as a network server is used to connect with the database from different JVMs across the network. We will start Java DB in embedded mode from the JDBCConnection.java
application. We will load the JDBC 4.0 driver automatically using the Java SE Service Provider mechanism. For automatic loading of the JDBC driver, we need to add the Java DB JDBC 4.0 driver JAR file, C:/Program Files/Sun/JavaDB/lib/derby.jar
to the CLASSPATH
variable. Java DB provides a batch script, setEmbeddedCP.bat
in the bin
directory to set the CLASSPATH
for the embedded mode. Run the setEmbeddedCP
script from the directory from which the JDBCConnection.java
application is to be run as follows:
"C:\Program Files\Sun\JavaDB\bin\setEmbeddedCP.bat"
JAR file, derby.jar
is added to the CLASSPATH
. The derby.jar
file includes a directory structure, META-INF/services/
, and a file, java.sql.Driver
, in the services
directory. The java.sql.Driver
file specifies the following JDBC driver class for the Java DB that is to be loaded automatically using the Java SE Service Provider mechanism:
org.apache.derby.jdbc.AutoloadedDriver
In the JDBCConnection.java
application, specify the connection URL for the Java DB database. Create a new database instance by specifying the database name, demodb
, and the create
attribute as, true
:
String url="jdbc:derby:demodb;create=true";
Connect with the Java DB database using the getConnection()
method of the DriverManager
class:
Connection conn = DriverManager.getConnection(url);
The DriverManager
automatically loads the JDBC 4.0 driver class, org.apache.derby.jdbc.AutoloadedDriver
, which is specified in the java.sql.Driver
file using the Java SE Service Provider mechanism. The JDBC driver is not required to be loaded using the Class.forName()
method using a JDBC 3.0 driver. The JDBCConnection.java
application is listed below:
import java.sql.*; public class JDBCConnection { public void connectToDatabase() { try { String url="jdbc:derby:demodb;create=true"; Connection conn = DriverManager.getConnection(url); System.out.println("Connection Established"); } catch (SQLException e) { System.out.println(e.getMessage()); } } public static void main(String[] argv) { JDBCConnection jdbc = new JDBCConnection(); jdbc.connectToDatabase(); } }
Other Relational Database Management Systems (RDBMS) databases provide a JDBC 4.0 driver that can be connected using the JDBC 4.0 driver, as discussed for the Java DB database. The connection URLs, and JDBC 4.0 drivers for some of the commonly used databases are discussed in following table:
